Introduction
These snippets compute and draw a graphic representation for the classical Mandelbrot set fractal.
Results
Running under pygame:
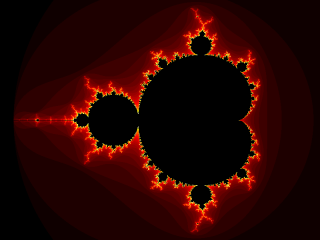
Running under a Nokia 770 with pymaemo (also pygame):
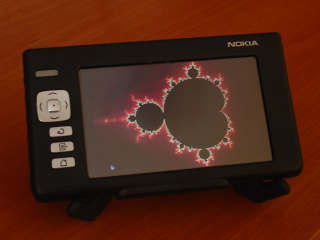
Running under a Nokia N70 with Python for Series 60:
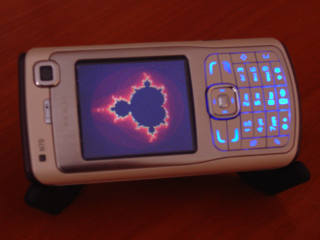
Code for pygame
1 from pygame.locals import *
2 import pygame
3
4 def main():
5 # Use 800, 480 with the Nokia 770
6 width, height = 800, 600
7 screen = pygame.display.set_mode((width,height),
8 HWSURFACE|DOUBLEBUF|FULLSCREEN)
9 xaxis = width/1.5
10 yaxis = height/2
11 scale = 250
12 iterations = 50
13 for y in range(height):
14 for x in range(width):
15 magnitude = 0
16 z = 0+0j
17 c = complex(float(x-xaxis)/scale, float(y-yaxis)/scale)
18 for i in range(iterations):
19 z = z**2+c
20 if abs(z) > 2:
21 v = 765*i/iterations
22 if v > 510:
23 color = (255, 255, v%255)
24 elif v > 255:
25 color = (255, v%255, 0)
26 else:
27 color = (v%255, 0, 0)
28 break
29 else:
30 color = (0, 0, 0)
31 screen.set_at((x, y), color)
32 pygame.display.update((0, y, width, 1))
33 event = pygame.event.poll()
34 if (event.type == QUIT or
35 (event.type == KEYDOWN and event.key == K_ESCAPE)):
36 return
37
38 while True:
39 event = pygame.event.poll()
40 if (event.type == QUIT or
41 (event.type == KEYDOWN and event.key == K_ESCAPE)):
42 break
43
44 if __name__ == "__main__":
45 main()
Code for Series 60
1 import appuifw
2 import e32
3
4 def main():
5 canvas = appuifw.Canvas()
6 appuifw.app.screen = 'full'
7 appuifw.app.body = canvas
8
9 width, height = canvas.size
10
11 xaxis = width/2
12 yaxis = height/1.5
13 scale = 60
14 iterations = 25
15 for y in range(height):
16 for x in range(width):
17 magnitude = 0
18 z = 0+0j
19 c = complex(float(y-yaxis)/scale, float(x-xaxis)/scale)
20 for i in range(iterations):
21 z = z**2+c
22 if abs(z) > 2:
23 v = 765*i/iterations
24 if v > 510:
25 color = (255, 255, v%255)
26 elif v > 255:
27 color = (255, v%255, 0)
28 else:
29 color = (v%255, 0, 0)
30 break
31 else:
32 color = (0, 0, 0)
33 canvas.point((x, y), color)
34 e32.ao_yield()
35
36 lock = e32.Ao_lock()
37 appuifw.app.exit_key_handler = lock.signal
38 lock.wait()
39
40 if __name__ == "__main__":
41 main()
Author
Gustavo Niemeyer <gustavo@niemeyer.net>